Permalink
Show file tree
Hide file tree
This commit does not belong to any branch on this repository, and may belong to a fork outside of the repository.
Showing
3,663 changed files
with
779,218 additions
and
0 deletions.
The diff you're trying to view is too large. We only load the first 3000 changed files.
There are no files selected for viewing
64
.settings
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,64 @@ | ||
[editor] | ||
tab_size = 2 | ||
; enter your editor preferences here... | ||
|
||
[ide] | ||
; enter your ide preferences here... | ||
|
||
[code-beautifier] | ||
; enter your code-beautifier preferences here... | ||
|
||
[codio-filetree] | ||
; enter your codio-filetree preferences here... | ||
|
||
[codio-lsp] | ||
; enter your codio-lsp preferences here... | ||
|
||
[junit] | ||
; enter your junit preferences here... | ||
|
||
[git] | ||
; enter your git preferences here... | ||
|
||
[terminal] | ||
; enter your terminal preferences here... | ||
|
||
[preview] | ||
; enter your preview preferences here... | ||
|
||
[emmet] | ||
; enter your emmet preferences here... | ||
|
||
[search] | ||
; enter your search preferences here... | ||
|
||
[guides] | ||
; enter your guides preferences here... | ||
|
||
[settings] | ||
; enter your settings preferences here... | ||
|
||
[account] | ||
; enter your account preferences here... | ||
|
||
[project] | ||
; enter your project preferences here... | ||
|
||
[install-software] | ||
; enter your install-software preferences here... | ||
|
||
[education] | ||
; enter your education preferences here... | ||
|
||
[deployment] | ||
; enter your deployment preferences here... | ||
|
||
[container] | ||
; enter your container preferences here... | ||
|
||
[sync-structure] | ||
; enter your sync-structure preferences here... | ||
|
||
[Codio Lexikon] | ||
; enter your Codio Lexikon preferences here... | ||
|
25
README.md
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,25 @@ | ||
### What is this? | ||
This `README.md` file is auto-created for all new projects. | ||
|
||
### Why am I here? | ||
This file opens automatically when you open a project. | ||
|
||
If you do not create Guides, this `README.md` will be what automatically opens for students. You can edit this file by clicking on the pencil icon in the upper right corner. | ||
|
||
### How do I get started with Codio? | ||
Use this [Onboarding Guide](https://codio.com/home/starter-packs/2ae8501b-e5f7-4b07-8e9f-adb155fc6d10) for an interactive tutorial through the main features of Codio. Click on the link, click **Use Pack** and then click **Create** to add it to your projects. | ||
|
||
### How do I close this file? | ||
At the top of your workspace you will see tabs for each open file. Click the x on the right hand side of the tab that says **README.md**. | ||
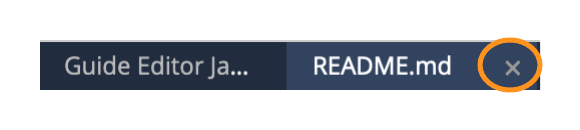 | ||
|
||
### I expected to see or edit learning materials. | ||
Select **Tools->Guide->Play** to view the Guide for this project. | ||
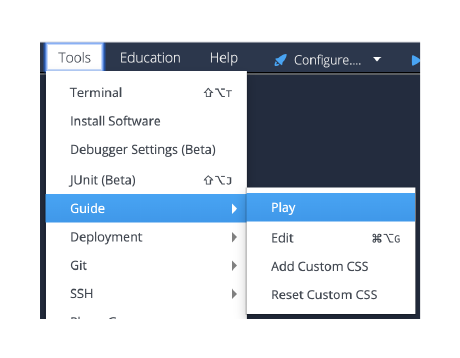 | ||
|
||
Click on the **Open Guides Editor** icon to edit the Guide. | ||
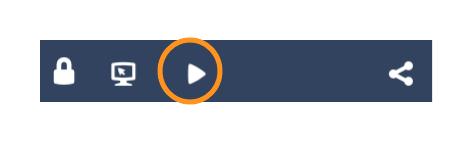 | ||
|
||
### How do I delete this file? | ||
To delete this `README.md` file, right-click (ctrl-click on a Mac) on the file name in the file list. | ||
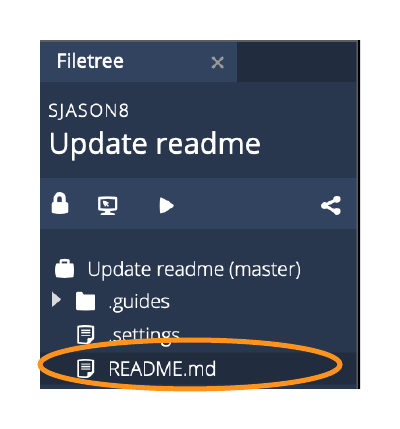 |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,137 @@ | ||
from flask import Flask | ||
import sqlite3 | ||
from flask import flash, session, render_template, request, redirect, url_for | ||
from werkzeug.security import generate_password_hash, check_password_hash | ||
|
||
con = sqlite3.connect('products.db') | ||
|
||
con.execute('CREATE TABLE products(id INT unsigned, name VARCHAR(255), code VARCHAR(255), image TEXT, price DOUBLE)') | ||
|
||
con.close() | ||
con = sqlite3.connect('products.db') | ||
|
||
con.execute('INSERT INTO products(id, name, code, image, price) VALUES (1, "American Tourist", "AMTR01", "product-images/bag.jpg", 12000.00),(2, "EXP Portable Hard Drive", "USB02", "product-images/external-hard-drive.jpg", 5000.00),(3, "Shoes", "SH03", "product-images/shoes.jpg", 1000.00),(4, "XP 1155 Intel Core Laptop", "LPN4", "product-images/laptop.jpg", 80000.00),(5, "FinePix Pro2 3D Camera", "3DCAM01", "product-images/camera.jpg", 150000.00),(6, "Simple Mobile", "MB06", "product-images/mobile.jpg", 3000.00),(7, "Luxury Ultra thin Wrist Watch", "WristWear03", "product-images/watch.jpg", 3000.00),(8, "Headphones", "HD08", "product-images/headphone.jpg", 400.00);') | ||
|
||
con.commit() | ||
con.close() | ||
|
||
|
||
|
||
|
||
app = Flask(__name__) | ||
app.secret_key = "secret key" | ||
|
||
@app.route('/add', methods=['POST']) | ||
def add_product_to_cart(): | ||
cursor = None | ||
try: | ||
_quantity = int(request.form['quantity']) | ||
_code = request.form['code'] | ||
|
||
if _quantity and _code and request.method == 'POST': | ||
con = sqlite3.connect('products.db') | ||
cur = con.cursor(); | ||
cur.execute("SELECT * FROM products WHERE code=?;", [_code]) | ||
row = cur.fetchone() | ||
itemArray = { row[2] : {'name' : row[1], 'code' : row[2], 'quantity' : _quantity, 'price' : row[4], 'image' : row[3], 'total_price': _quantity * row[4]}} | ||
print('itemArray is', itemArray) | ||
|
||
all_total_price = 0 | ||
all_total_quantity = 0 | ||
|
||
session.modified = True | ||
|
||
if 'cart_item' in session: | ||
print('in session') | ||
if row[2] in session['cart_item']: | ||
for key, value in session['cart_item'].items(): | ||
if row[2] == key: | ||
old_quantity = session['cart_item'][key]['quantity'] | ||
total_quantity = old_quantity + _quantity | ||
session['cart_item'][key]['quantity'] = total_quantity | ||
session['cart_item'][key]['total_price'] = total_quantity * row[4] | ||
else: | ||
session['cart_item'] = array_merge(session['cart_item'], itemArray) | ||
|
||
for key, value in session['cart_item'].items(): | ||
individual_quantity = int(session['cart_item'][key]['quantity']) | ||
individual_price = float(session['cart_item'][key]['total_price']) | ||
all_total_quantity = all_total_quantity + individual_quantity | ||
all_total_price = all_total_price + individual_price | ||
else: | ||
session['cart_item'] = itemArray | ||
all_total_quantity = all_total_quantity + _quantity | ||
all_total_price = all_total_price + _quantity * row[4] | ||
|
||
session['all_total_quantity'] = all_total_quantity | ||
session['all_total_price'] = all_total_price | ||
|
||
return redirect(url_for('.products')) | ||
else: | ||
return 'Error while adding item to cart' | ||
except Exception as e: | ||
print(e) | ||
finally: | ||
cur.close() | ||
con.close() | ||
|
||
@app.route('/') | ||
def products(): | ||
try: | ||
con = sqlite3.connect('products.db') | ||
cur = con.cursor(); | ||
cur.execute("SELECT * FROM products") | ||
rows = cur.fetchall() | ||
return render_template('products.html', products=rows) | ||
except Exception as e: | ||
print(e) | ||
finally: | ||
cur.close() | ||
con.close() | ||
|
||
@app.route('/empty') | ||
def empty_cart(): | ||
try: | ||
session.clear() | ||
return redirect(url_for('.products')) | ||
except Exception as e: | ||
print(e) | ||
|
||
@app.route('/delete/<string:code>') | ||
def delete_product(code): | ||
try: | ||
all_total_price = 0 | ||
all_total_quantity = 0 | ||
session.modified = True | ||
|
||
for item in session['cart_item'].items(): | ||
if item[0] == code: | ||
session['cart_item'].pop(item[0], None) | ||
if 'cart_item' in session: | ||
for key, value in session['cart_item'].items(): | ||
individual_quantity = int(session['cart_item'][key]['quantity']) | ||
individual_price = float(session['cart_item'][key]['total_price']) | ||
all_total_quantity = all_total_quantity + individual_quantity | ||
all_total_price = all_total_price + individual_price | ||
break | ||
|
||
if all_total_quantity == 0: | ||
session.clear() | ||
else: | ||
session['all_total_quantity'] = all_total_quantity | ||
session['all_total_price'] = all_total_price | ||
return redirect(url_for('.products')) | ||
except Exception as e: | ||
print(e) | ||
|
||
def array_merge( first_array , second_array ): | ||
if isinstance( first_array , list ) and isinstance( second_array , list ): | ||
return first_array + second_array | ||
elif isinstance( first_array , dict ) and isinstance( second_array , dict ): | ||
return dict( list( first_array.items() ) + list( second_array.items() ) ) | ||
elif isinstance( first_array , set ) and isinstance( second_array , set ): | ||
return first_array.union( second_array ) | ||
return False | ||
|
||
if __name__ == "__main__": | ||
app.run() |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,124 @@ | ||
body { | ||
font-family: Arial; | ||
color: #211a1a; | ||
font-size: 0.9em; | ||
} | ||
|
||
#shopping-cart { | ||
margin: 40px; | ||
} | ||
|
||
#product-grid { | ||
margin: 40px; | ||
} | ||
|
||
#shopping-cart table { | ||
width: 100%; | ||
background-color: #F0F0F0; | ||
} | ||
|
||
#shopping-cart table td { | ||
background-color: #FFFFFF; | ||
} | ||
|
||
.txt-heading { | ||
color: #211a1a; | ||
border-bottom: 1px solid #E0E0E0; | ||
overflow: auto; | ||
} | ||
|
||
#btnEmpty { | ||
background-color: #ffffff; | ||
border: #d00000 1px solid; | ||
padding: 5px 10px; | ||
color: #d00000; | ||
float: right; | ||
text-decoration: none; | ||
border-radius: 3px; | ||
margin: 10px 0px; | ||
} | ||
|
||
.btnAddAction { | ||
padding: 5px 10px; | ||
margin-left: 5px; | ||
background-color: #efefef; | ||
border: #E0E0E0 1px solid; | ||
color: #211a1a; | ||
float: right; | ||
text-decoration: none; | ||
border-radius: 3px; | ||
cursor: pointer; | ||
} | ||
|
||
#product-grid .txt-heading { | ||
margin-bottom: 18px; | ||
} | ||
|
||
.product-item { | ||
float: left; | ||
background: #ffffff; | ||
margin: 30px 30px 0px 0px; | ||
border: #E0E0E0 1px solid; | ||
} | ||
|
||
.product-image { | ||
height: 155px; | ||
width: 250px; | ||
background-color: #FFF; | ||
} | ||
|
||
.clear-float { | ||
clear: both; | ||
} | ||
|
||
.demo-input-box { | ||
border-radius: 2px; | ||
border: #CCC 1px solid; | ||
padding: 2px 1px; | ||
} | ||
|
||
.tbl-cart { | ||
font-size: 0.9em; | ||
} | ||
|
||
.tbl-cart th { | ||
font-weight: normal; | ||
} | ||
|
||
.product-title { | ||
margin-bottom: 20px; | ||
} | ||
|
||
.product-price { | ||
float:left; | ||
} | ||
|
||
.cart-action { | ||
float: right; | ||
} | ||
|
||
.product-quantity { | ||
padding: 5px 10px; | ||
border-radius: 3px; | ||
border: #E0E0E0 1px solid; | ||
} | ||
|
||
.product-tile-footer { | ||
padding: 15px 15px 0px 15px; | ||
overflow: auto; | ||
} | ||
|
||
.cart-item-image { | ||
width: 30px; | ||
height: 30px; | ||
border-radius: 50%; | ||
border: #E0E0E0 1px solid; | ||
padding: 5px; | ||
vertical-align: middle; | ||
margin-right: 15px; | ||
} | ||
.no-records { | ||
text-align: center; | ||
clear: both; | ||
margin: 38px 0px; | ||
} |
Sorry, something went wrong. Reload?
Sorry, we cannot display this file.
Sorry, this file is invalid so it cannot be displayed.
Sorry, something went wrong. Reload?
Sorry, we cannot display this file.
Sorry, this file is invalid so it cannot be displayed.
Sorry, something went wrong. Reload?
Sorry, we cannot display this file.
Sorry, this file is invalid so it cannot be displayed.
Sorry, something went wrong. Reload?
Sorry, we cannot display this file.
Sorry, this file is invalid so it cannot be displayed.
Sorry, something went wrong. Reload?
Sorry, we cannot display this file.
Sorry, this file is invalid so it cannot be displayed.
Sorry, something went wrong. Reload?
Sorry, we cannot display this file.
Sorry, this file is invalid so it cannot be displayed.
Sorry, something went wrong. Reload?
Sorry, we cannot display this file.
Sorry, this file is invalid so it cannot be displayed.
Sorry, something went wrong. Reload?
Sorry, we cannot display this file.
Sorry, this file is invalid so it cannot be displayed.
Oops, something went wrong.